Provide tailored mobile solutions with our MVNX API
Use our APIs to create unique, customized mobile services that cater to your consumer market .
Get StartedEndpoints
Request
const fetch = require('node-fetch');
const url = 'https://lgw.att.com/InquireSubscriberProfile';
const options = {
method: 'POST',
headers: {accept: 'application/json', 'content-type': 'application/json'},
body: JSON.stringify({
MessageHeader: {
TrackingMessageHeader: {
version: '280',
messageId: 'occaecat',
timeToLive: 57279159,
originatorId: 'ut sunt officia',
dateTimeStamp: '1957-03-11T05:23:58.0Z',
conversationId: 'quis adipisicing culpa ipsum ullamco',
applicationName: 'CustomerCareProfile',
originalVersion: 'ut non ad qui aliqua',
uniqueTransactionId: 'elit et',
infrastructureVersion: '86',
originalInfrastructureVersion: 'sunt quis'
},
SecurityMessageHeader: {userName: 'anim', userPassword: 'reprehenderit tempor consectetur sunt'},
SequenceMessageHeader: {sequenceNumber: 'ipsum', totalInSequence: 'nulla'}
},
InquireSubscriberProfileRequest: {
est96: -68846629,
ide: false,
Duis_328: true,
laboris433: true,
ut_2_: 28537591.381988928,
et07: true,
inb3d: 'commodo eiusmod tempor mollit',
anim_5: -18400210,
Duis3: false,
occaecat_5: true,
dolor75: true,
sit_9c: 56253706.98897281,
consequat_7: -31976036.39436473,
minimff: 66336534.688994974,
officiacdb: 14185842
}
})
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
Response
{
"MessageHeader": {
"TrackingMessageHeader": {
"version": "280",
"messageId": "devextest08172023",
"timeToLive": 300000,
"dateTimeStamp": "2023-08-17T01:14:12Z",
"conversationId": "XXXXXXXXXXXXXX~CNG-CSI~aba037f4-cc1c-448b-8f56-9944b0ed2e2e",
"applicationName": "CustomerCareProfile",
"uniqueTransactionId": "ServiceGateway321838@qzlty22047c01_d183474c-f5aa-49a4-962b-a1e1d19af323",
"infrastructureVersion": "86"
},
"SecurityMessageHeader": {
"userName": "[userName]",
"userPassword": "[userPassword]"
},
"SequenceMessageHeader": {
"sequenceNumber": "1",
"totalInSequence": "1"
}
},
"InquireSubscriberProfileResponse": {
"Response": {
"code": "0",
"description": "Success"
},
"Subscriber": {
"LTE": {
"imsHSSId": "IH2",
"imsCPMId": "CPM2"
},
"hlrId": "DUM",
"deposit": {
"subscriberDepositAmount": 0
},
"Contract": {
"reasonCode": "PPD",
"ContractTerm": {
"term": 0,
"Commission": {
"dealer": {
"code": "XH8YB",
"secondaryCode": "XH8YB"
},
"salesRepresentative": "XH8YB"
}
}
},
"epcHSSId": "HS3",
"PricePlan": {
"Commission": {
"dealer": {
"code": "XH8YB"
},
"location": "XH8YB",
"salesRepresentative": "XH8YB"
},
"description": "REACH MOBILE R9DA",
"singleUserCode": "R9DA",
"EffectiveDates": {
"effectiveDate": "2023-08-07"
},
"recurringCharge": 0,
"OfferingsBundled": [
{
"action": "Q",
"offeringCode": "BVMRPO",
"offeringDescription": "BasicVM-MssgNotif PPD",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "BVM2",
"featureDescription": "Basic Voice Mail"
},
{
"featureCode": "VMWI",
"featureDescription": "Message Waiting Ind"
}
]
},
{
"action": "Q",
"offeringCode": "R9DAMMSIO",
"offeringDescription": "R9DA OPT MMS",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "MMIDUM",
"featureDescription": "NOPROVISIONINGMMS"
},
{
"featureCode": "MMR1",
"featureDescription": "GENERICMMSPROFILE1",
"dataUsageCategory": "MMS"
}
]
},
{
"action": "Q",
"offeringCode": "R9DARDS2O",
"offeringDescription": "R9DA OPT DATA RATING",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "5GPRO",
"featureDescription": "5G Provisioning"
},
{
"featureCode": "HSSBRD",
"featureDescription": "HSS PROVISIONING"
},
{
"featureCode": "LTECOS",
"featureDescription": "LTECOS"
},
{
"featureCode": "POST",
"featureDescription": "POST POLICY"
},
{
"featureCode": "R5GAPN",
"featureDescription": "Reseller 5G APN",
"dataUsageCategory": "GPRS"
},
{
"featureCode": "RM42G",
"featureDescription": "RM42G"
}
],
"category": "CCS"
},
{
"action": "Q",
"offeringCode": "R9DASMSIO",
"offeringDescription": "R9DA OPT SMS",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "SMR6",
"featureDescription": "SMSPROFILE6",
"dataUsageCategory": "SMS"
},
{
"featureCode": "SMSI",
"featureDescription": "Int'l Text Messaging"
}
]
},
{
"action": "Q",
"offeringCode": "RMABRO",
"offeringDescription": "RM OPT ABR SOC",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "RMABR",
"featureDescription": "RMABR"
}
]
},
{
"action": "Q",
"offeringCode": "RMELA",
"offeringDescription": "RM OPT ONNET PPD",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "ELACA",
"featureDescription": "Dummy Feature For ELA Soc (Calling Areas)"
}
]
},
{
"action": "Q",
"offeringCode": "RMELAT",
"offeringDescription": "RM O ONNET PLS TOLL",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "ELATCA",
"featureDescription": "Extended Local Area Including Toll(Calling Areas)"
}
]
},
{
"action": "Q",
"offeringCode": "RMHMLD",
"offeringDescription": "RM OPT HOME TOLL",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "TDOMES",
"featureDescription": "Toll Domestic"
}
]
},
{
"action": "Q",
"offeringCode": "RMHRLD",
"offeringDescription": "RM OPT ROAM TOLL",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "TDOMES",
"featureDescription": "Toll Domestic"
}
]
},
{
"action": "Q",
"offeringCode": "RMHSO",
"offeringDescription": "RM O HOTSPOT SOC",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "GTETH",
"featureDescription": "Mob Tethering"
},
{
"featureCode": "LMHS1",
"featureDescription": "Hot Spot 1 to 1"
},
{
"featureCode": "LMHSM",
"featureDescription": "Hot Spot 1 to Many"
},
{
"featureCode": "LTETH",
"featureDescription": "Mob Tethering"
},
{
"featureCode": "RSMHT",
"featureDescription": "Resellermht APN HSMX",
"dataUsageCategory": "GPRS"
}
],
"category": "CCS"
},
{
"action": "Q",
"offeringCode": "RMILDCO",
"offeringDescription": "RM O INTL CALL ALLOW",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "ILDS",
"featureDescription": "IntLongDistAllowed"
}
]
},
{
"action": "Q",
"offeringCode": "RMROCSTRK",
"offeringDescription": "RM OPT OCS TRACKING",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "OCSTRK",
"featureDescription": "Enabler OCS Trk"
}
]
},
{
"action": "Q",
"offeringCode": "RMRZ1O",
"offeringDescription": "OPT US ROAM ZONE 1",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "RMRZ1",
"featureDescription": "US ROAM ZONE 1"
},
{
"featureCode": "RSLDC",
"featureDescription": "RSL Data Rm Spd Cntl"
}
]
},
{
"action": "Q",
"offeringCode": "RMVLTEO",
"offeringDescription": "RM OPT VOLTE SOC",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "IMS",
"featureDescription": "Data Pay Per Use"
},
{
"featureCode": "VCTS",
"featureDescription": "ALU vCTS Migration"
},
{
"featureCode": "VOLTE",
"featureDescription": "Voice over LTE"
}
],
"category": "CCS"
},
{
"action": "Q",
"offeringCode": "R9DA",
"offeringDescription": "REACH MOBILE R9DA",
"OfferingEffectiveBillDates": {
"startDate": "2023-08-07"
},
"OfferingFeatures": [
{
"featureCode": "CFC",
"featureDescription": "Call Forward Conditional"
},
{
"featureCode": "CFI",
"featureDescription": "Call Forward Immediate"
},
{
"featureCode": "CH",
"featureDescription": "Call Hold"
},
{
"featureCode": "CLI",
"featureDescription": "Caller ID"
},
{
"featureCode": "CW",
"featureDescription": "Call Waiting"
},
{
"featureCode": "EP840",
"featureDescription": "EP840"
},
{
"featureCode": "MPTY",
"featureDescription": "6 Way Calling"
},
{
"featureCode": "QD",
"featureDescription": "Direct Bill Detail"
}
]
}
],
"RestrictedSubMarkets": [
{
"code": "ALL"
}
],
"ratePlanGenAttribute": "RTP;BVM;"
},
"defaultAPN": "ERESELLER",
"Commission": {
"dealer": {
"code": "XH8YB"
},
"location": "XH8YB",
"salesRepresentative": "XH8YB"
},
"voiceMailId": "DCS5",
"productType": "G",
"SpendingLimit": {
"status": "S"
},
"BillingMarket": {
"billingMarket": "GAC",
"billingSubMarket": "708"
},
"depositAmount": 0,
"subscriptionID": "T_GAC_708_1691408858000244422868",
"subscriberNumber": "8035616216",
"SubscriberStatus": {
"statusDate": "2023-08-30",
"subscriberStatus": "S",
"statusReasonCode": "OT",
"isSuspensionVoluntary": true
},
"deviceInformation": {
"device": [
{
"equipmentType": "G",
"technologyType": "LTE",
"IMSI": "310410800361478",
"IMEI": "353039090000002",
"IMEIType": "J9",
"SIM": "89014103278003614784",
"Manufacturer": {
"make": "APPLE",
"model": "iPhoXA1901"
},
"umtsCapability": true,
"nfcCapability": "N",
"pinUnblockingCode1": "94651274",
"pinUnblockingCode2": "43580113",
"effectiveDate": "2023-08-07",
"lteType": "7",
"IMSIPublicId": "310410800361478@one.att.net",
"smsCapabilityIndicator": true,
"pcCardIndicator": false,
"uiccCode": "B",
"uiccKeys": {
"publicKey": [
"8035616216@one.att.net"
],
"privateKey": "310410800361478@private.att.net"
},
"certificationStatus": true
}
]
},
"ContactInformation": {
"Name": {
"lastName": "REACHNTCC",
"firstName": ""
},
"PpuAddress": {
"Zip": {
"zipCode": "29201"
},
"City": "COLUMBIA",
"State": "SC",
"AddressLine1": "400 LAUREL ST"
}
},
"AdditionalOfferings": [
{
"action": "Q",
"offeringCode": "NC001",
"offeringDescription": "NC001 SUBCLASS ID",
"Commission": {
"dealer": {
"code": "XH8YB",
"secondaryCode": "XH8YB"
},
"location": "XH8YB",
"salesRepresentative": "XH8YB"
},
"OfferingFeatures": [
{
"featureCode": "NC001",
"featureDescription": "NC001 SUBCLASS ID"
}
],
"EffectiveDates": {
"effectiveDate": "2023-08-07"
},
"oneTimeCharge": 0,
"sequenceNumber": 2661092317,
"category": "RTP;DSB_ALLOW;RSLR_SUBCLASS;",
"genAttribute": "RTP;DSB_ALLOW;RSLR_SUBCLASS;",
"delayRePriceIndicator": false
},
{
"action": "Q",
"offeringCode": "ALUVCTS",
"offeringDescription": "ALU vCTS tracking",
"Commission": {
"dealer": {
"code": "XH8YB",
"secondaryCode": "XH8YB"
},
"location": "XH8YB",
"salesRepresentative": "XH8YB"
},
"OfferingFeatures": [
{
"featureCode": "VCTS",
"featureDescription": "ALU vCTS Migration"
}
],
"EffectiveDates": {
"effectiveDate": "2023-08-07"
},
"oneTimeCharge": 0,
"sequenceNumber": 2661092318,
"category": "RESSOCREM;SKIP_SOC_MATCH;",
"genAttribute": "RESSOCREM;SKIP_SOC_MATCH;",
"delayRePriceIndicator": false
}
],
"longDistanceCarrier": "5475",
"subscriberIndicators": {
"churn": 0,
"pttFeature": false,
"cseRatePlan": false,
"profitability": 0,
"vmPlatformType": "B",
"smsFeatureIndicator": true,
"gaitFeatureIndicator": false,
"portabilityIndicator": true,
"pendingEquipmentUpgrade": false
},
"billingAccountNumber": "534198059455",
"ServiceActivationDate": {
"effectiveDate": "2023-08-07"
},
"crossUpgradeIndicator": "false",
"SolicitationIndicators": [
{
"solicitationMedium": "EMAIL",
"enabled": false
},
{
"solicitationMedium": "MAIL",
"enabled": false
},
{
"solicitationMedium": "PHONE",
"enabled": false
},
{
"solicitationMedium": "SMS",
"enabled": false
}
],
"MobileToAnyNumberDetails": {
"isEffective": false,
"callListCount": 0,
"isHistoryAvailable": false,
"maxNumberOfCallList": 0,
"minPricePlanRecurringCharge": 0
}
}
}
}
Tailored plans
Target niche markets with tailored plans and services to cater to specific needs. Tailored plans can target consumer groups such as families, students, seniors or travelers.
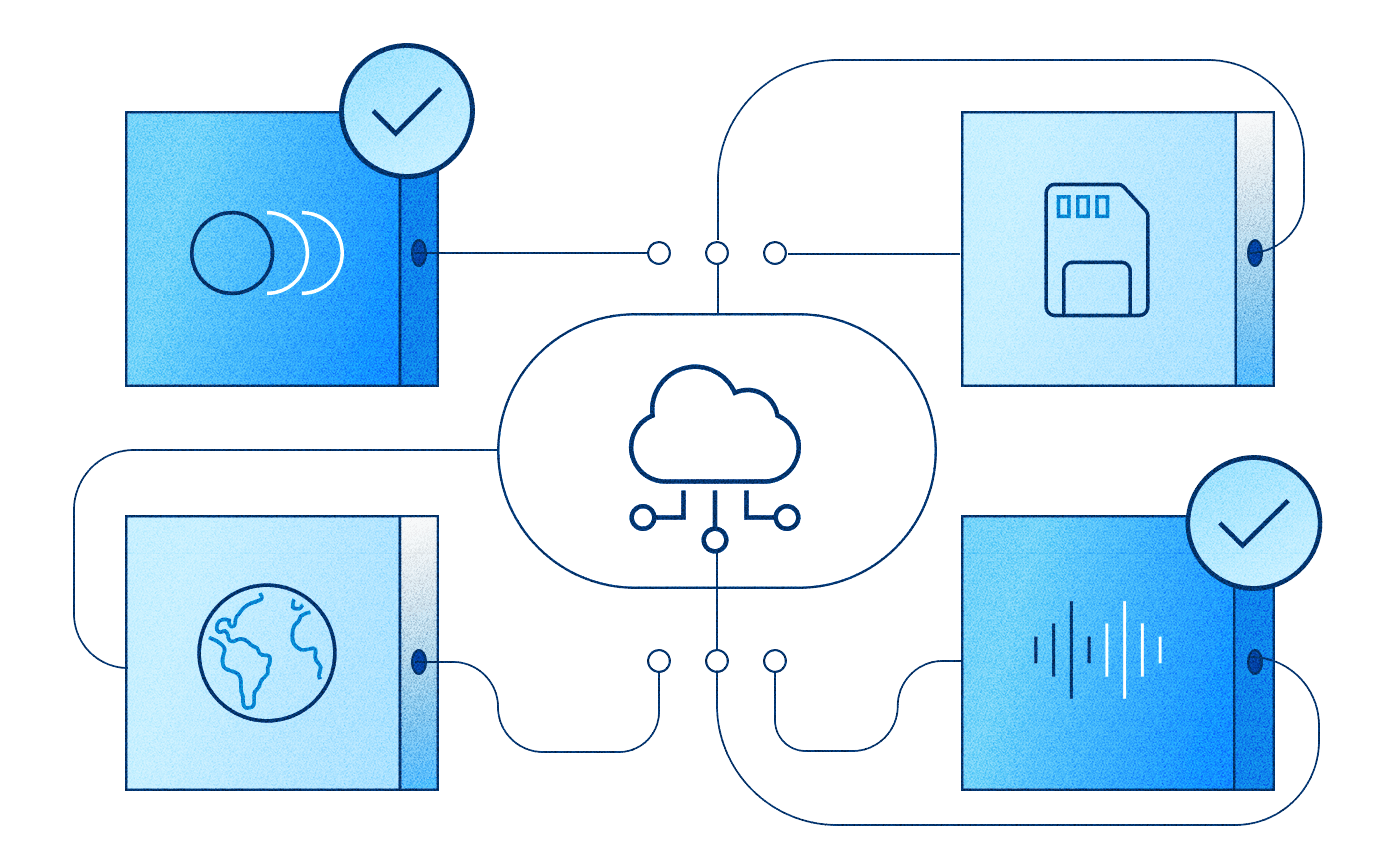
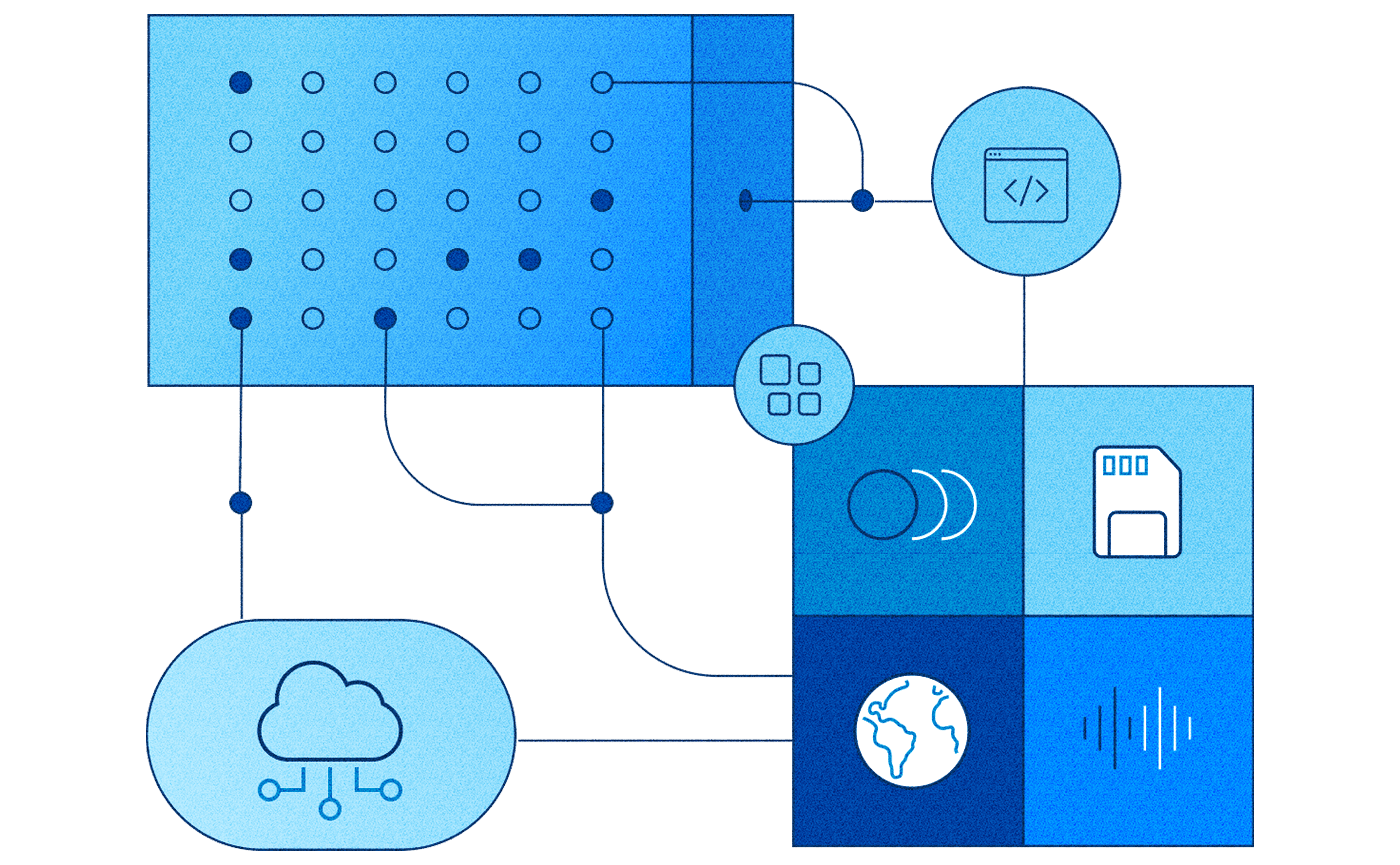
Bundled services
Bundle your mobile services with other products such as home internet or home security, to provide your customers with a full range of networking solutions.
Bring your own device (BYOD)
Allow your customers to use their existing devices and phone numbers while using your enhanced carrier services.
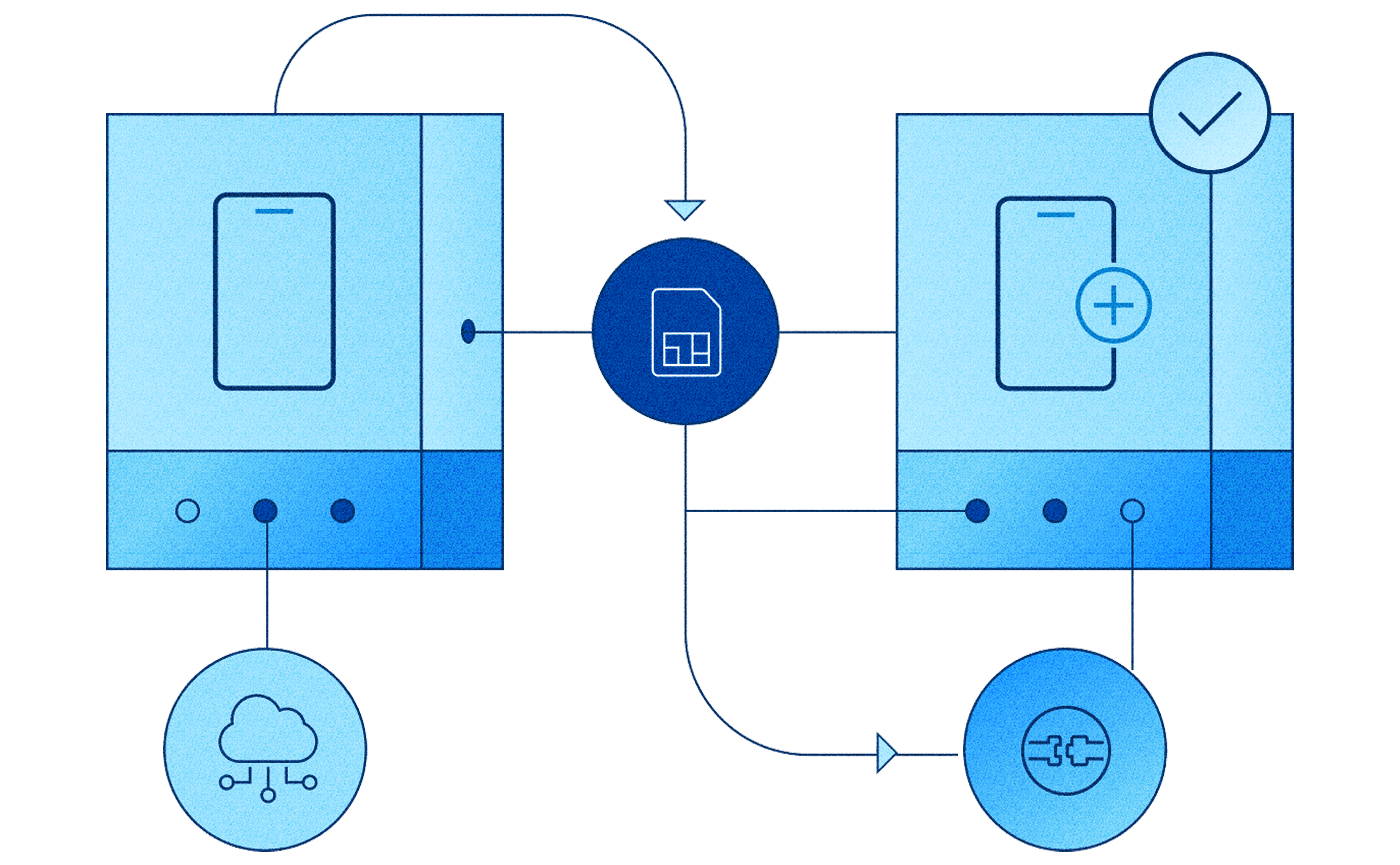
MVNX APIs
Our RESTful APIs make creating customer solutions a snap. With our APIs you can hand-tailor a wide range of business offerings.
Data-only plans
Help customers who primarily use their devices for internet access
Emergency services
Offer a network dedicated to emergency services such as alerts and public safety notifications
Niche markets
Provide services and plans tailored to the senior market
Get started with AT&T MVNX APIs
With our APIs you can hand-tailor a wide range of business offerings.